Setting up EasyAR iOS SDK¶
This article shows how to setup EasyAR non-Unity iOS projects using EasyAR package.
If you want to use EasyAR unity package, please read this for setup and this for samples.
If you want to run EasyAR non-Unity iOS sample, please read this instead.
Pre-Requirements¶
XCode 6 or later (we have tested in XCode 6.4 and XCode 7.1)
iPhone or iPad device, or other real Apple devices (EasyAR do not support running on the simulator)
Static framework or dynamic framework¶
Static framework and dynamic framework are not official words from Apple. We use these phrases for convenience, to represent framework with static libraries and framework with dynamic libraries.
EasyAR SDK provides both static framework and dynamic framework from 2.0. Only static frameworks are provided in EasyAR SDK 1.x versions.
Dynamic framework is brought into iOS since iOS 8.0 and you should target iOS 8 or later in your app when using dynamic framework.
If you are not familiar with Apple's dynamic framework, you can learn how to Embedding Frameworks In An App from Apple.
Some hints for choosing the framework,
Dynamic framework cannot target for iOS 7, so if you are willing to have iOS 7 compatibility for your app, use static framework.
Apple limits executable binary size when uploading to app store, so if you have a very large executable, you can choose dynamic framework, which will not increase the size of executable. Please notice that the total size of app will not change.
Execution time, performance or features will not be different when using different type of frameworks.
Add Frameworks¶
Static Framework¶
You need to add these frameworks when using EasyAR static framework.
easyar.framework
libc++.tbd
AVFoundation.framework
CoreGraphics.framework
CoreImage.framework
CoreMedia.framework
CoreVideo.framework
OpenGLES.framework
UIKit.framework
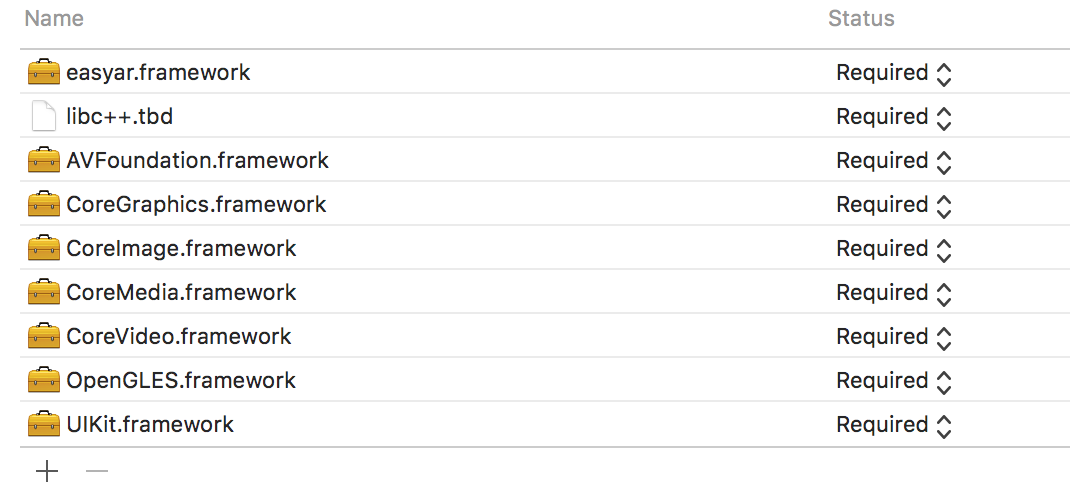
For EasyAR SDK Pro, you need these additional frameworks
Accelerate.framework
CoreMotion.framework
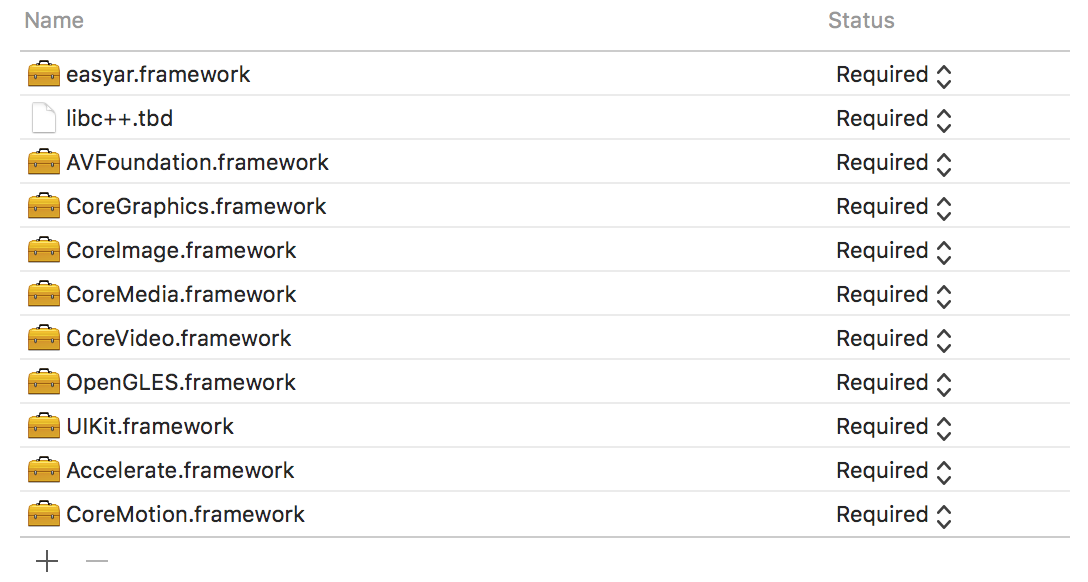
Dynamic Framework¶
Only easyar.framework is required when using EasyAR dynamic framework.
easyar.framework
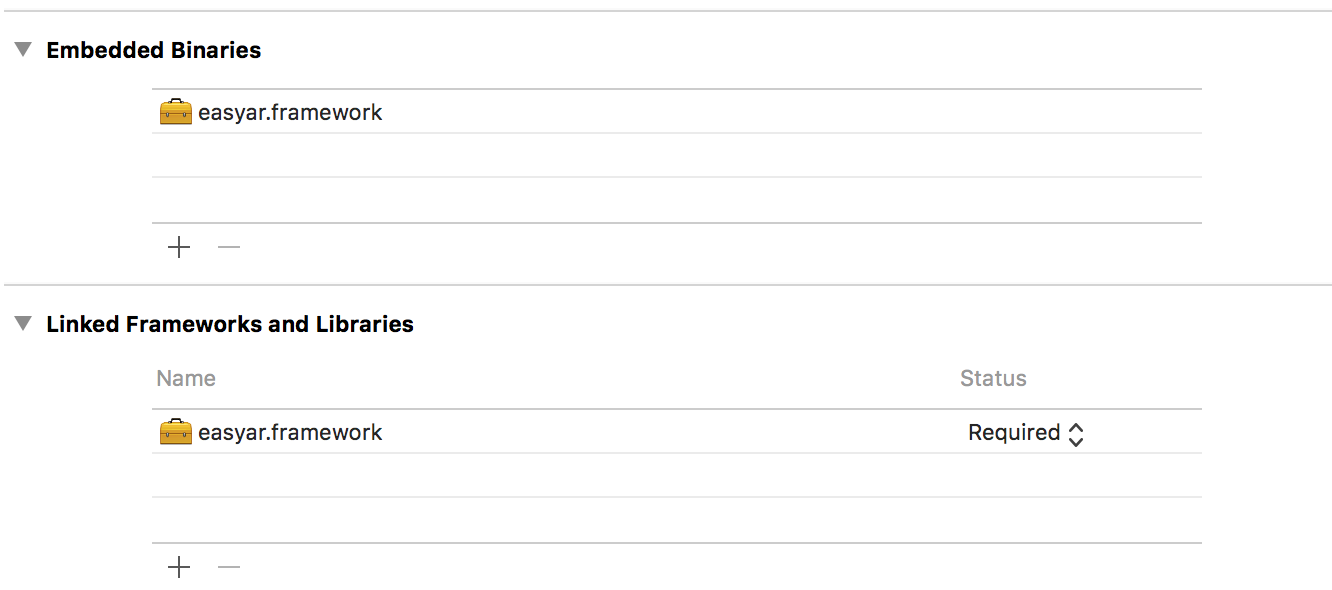
Do not forget to add easyar.framework in the Embedded Binaries list.
Disable Bitcode¶
EasyAR do not use bitcode and EasyAR do not offer bitcode compatibility.
Make sure to disable bitcode in your build settings,
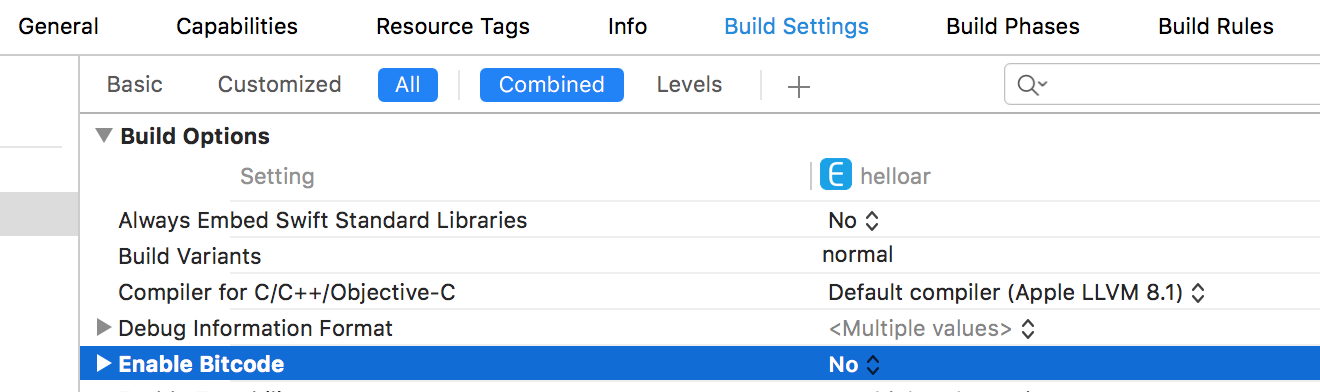
Initialize EasyAR¶
Use initialize: method of easyar_Engine to initialize EasyAR. You can add the initialize into your code like this.
[easyar_Engine initialize:key];
Set rotation¶
Use setRotation: method of easyar_Engine to set rotation. You can add the code like this.
-(void)willRotateToInterfaceOrientation:(UIInterfaceOrientation)toInterfaceOrientation duration:(NSTimeInterval)duration{
[super willRotateToInterfaceOrientation:toInterfaceOrientation duration:duration];
switch (toInterfaceOrientation)
{
case UIInterfaceOrientationPortrait:
[easyar_Engine setRotation:270];
break;
case UIInterfaceOrientationPortraitUpsideDown:
[easyar_Engine setRotation:90];
break;
case UIInterfaceOrientationLandscapeLeft:
[easyar_Engine setRotation:180];
break;
case UIInterfaceOrientationLandscapeRight:
[easyar_Engine setRotation:0];
break;
default:
break;
}
}
Privacy Settings¶
Some privacy settings are required.
To use camera, add Privacy - Camera Usage Description,

To use screen recording feature, add Privacy - Microphone Usage Description,

Other Code¶
The rest is to write EasyAR logics and other code. You can reference EasyAR samples for more details.
How to use Swift API¶
EasyAR SDK Swift API is introduced in 2.1.0, if you want to use the built-in Swift API, you have to use EasyAR SDK 2.1.0 or later versions.
EasyAR SDK Swift API is provided in source code for the best compatibility as Apple do not provide a strong ABI across different Swift versions.
To use EasyAR SDK Swift API, you need to create a framework project and embed the generated framework target into your own project. EasyAR do not provide a pre-configured XCode project for this, because project settings may vary from different projects.
Create EasyARSwift framework project¶
Create a new project of type Cocoa Touch Framework and name it as EasyARSwift
You can choose to embed EasyARSwift project into your app project or create a sperate project.
Import EasyAR Swift code into EasyARSwift project
The EasyARSwift.h generated automatically by XCode is useless here, it is safe to remove it.
Setup Objective-C Bridging Header in build settings
Please note this option will not show in XCode before you import any Swift files into the project, so make sure to import swift code first.
Import static easyar.framework into EasyARSwift project for link
Add Linked Frameworks and Librarires
You need to add these frameworks when using EasyAR static framework.
easyar.framework
libc++.tbd
AVFoundation.framework
CoreGraphics.framework
CoreImage.framework
CoreMedia.framework
CoreVideo.framework
OpenGLES.framework
UIKit.framework
For EasyAR SDK Pro, you need these additional frameworks
Accelerate.framework
CoreMotion.framework
Disable Bitcode
EasyAR do not use bitcode and EasyAR do not offer bitcode compatibility.
Make sure to disable bitcode in your build settings,
Deployment Target
Change deployment target to fit your app project, make sure it is lower than or equal to your app project.
Embedding and use EasyARSwift framework¶
Embedding EasyARSwift framework into your project
import EasyARSwift in Swift source code
You can reference HelloARSwift sample code or API reference for how to use.