Setting up EasyAR Unity SDK¶
This article shows how to setup EasyAR Unity projects using EasyAR Unity package.
If you want to run EasyAR unity sample, please read this instead.
Pre-Requirements¶
Unity 4.6 or later
(If target for Android) Android SDK with Build Tools at least version 23.0.1
(If target for iOS) iPhone or iPad device, or other real Apple devices (EasyAR do not support running on the simulator)
Import Package¶
First, you have to download EasyAR Unity package (.unitypackage) and open to import it into Unity.
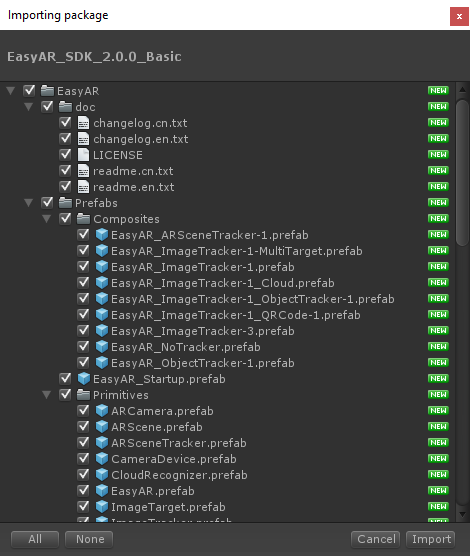
Initialize EasyAR¶
To make EasyAR work, you have to have EasyAR prefab and/or other prefabs in the scene. There is an EasyAR_Startup Prefab for general use. Drag EasyAR_Startup Prefab or prefabs you need into the scene.
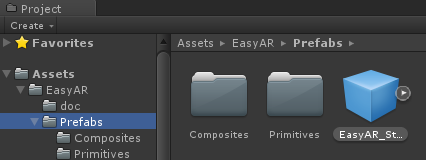
Input key on the inspector.
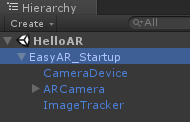
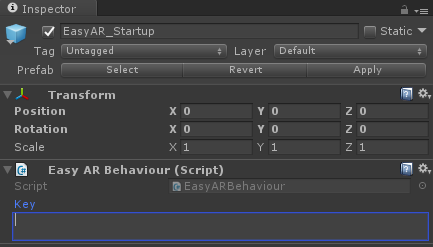
You can create a key after login EasyAR website. You can find how to create the key from Getting Started with EasyAR SDK.
Add Target¶
Planar Image Tracking¶
You need ImageTarget when using planar image tracking.
There are many ways to use the ImageTarget. You can reference the HelloARTarget sample.
If you want to setup ImageTarget statically in the scene, you have to drag an ImageTarget Prefab into the scene. Read ImageTarget Prefab and ImageTargetBaseBehaviour in the Reference for Unity for more details.
Object under the target node should be bottom aligned with ImageTarget,
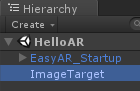
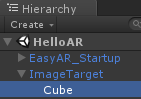
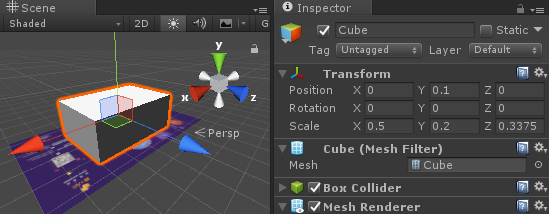
3D Object Tracking¶
You need ObjectTarget when using 3D object tracking. It is similar with ImageTarget.
Rotation of object under the ObjectTarget should be set to,
X: 90
y: 180
z: 0
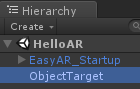
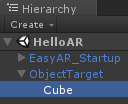
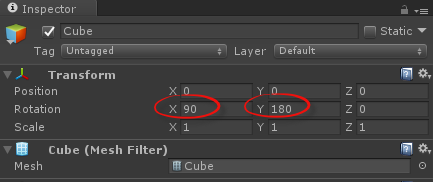
SLAM¶
You need ARSceneTarget when using SLAM. It is similar with ImageTarget.
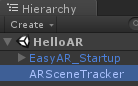
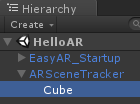
Target Events¶
Different type of targets use similar events. For ImageTarget and ObjectTarget, there are found/lost and load/unload events. ARSceneTarget has only found/lost events.
Here we introduce only events for ImageTarget, you can get events for other type of targets by replacing the target type in below code. You can also reference samples.
For ImageTarget, You can handle target event either in ImageTargetBehaviour,
public class EasyImageTargetBehaviour : ImageTargetBehaviour
{
protected override void Awake()
{
base.Awake();
TargetFound += OnTargetFound;
TargetLost += OnTargetLost;
TargetLoad += OnTargetLoad;
TargetUnload += OnTargetUnload;
}
void OnTargetFound(TargetAbstractBehaviour behaviour)
{
Debug.Log("Found: " + Target.Id);
}
void OnTargetLost(TargetAbstractBehaviour behaviour)
{
Debug.Log("Lost: " + Target.Id);
}
void OnTargetLoad(ImageTargetBaseBehaviour behaviour, ImageTrackerBaseBehaviour tracker, bool status)
{
Debug.Log("Load target (" + status + "): " + Target.Id + " (" + Target.Name + ") " + " -> " + tracker);
}
void OnTargetUnload(ImageTargetBaseBehaviour behaviour, ImageTrackerBaseBehaviour tracker, bool status)
{
Debug.Log("Unload target (" + status + "): " + Target.Id + " (" + Target.Name + ") " + " -> " + tracker);
}
}
or in a global target manager,
public class ARIsEasyBehaviour : MonoBehaviour
{
private void Awake()
{
var EasyARBehaviour = FindObjectOfType<EasyARBehaviour>();
EasyARBehaviour.Initialize();
foreach (var behaviour in ARBuilder.Instance.ARCameraBehaviours)
{
behaviour.TargetFound += OnTargetFound;
behaviour.TargetLost += OnTargetLost;
}
foreach (var behaviour in ARBuilder.Instance.ImageTrackerBehaviours)
{
behaviour.TargetLoad += OnTargetLoad;
behaviour.TargetUnload += OnTargetUnload;
}
}
void OnTargetFound(ARCameraBaseBehaviour arcameraBehaviour, TargetAbstractBehaviour targetBehaviour, Target target)
{
Debug.Log("<Global Handler> Found: " + target.Id);
}
void OnTargetLost(ARCameraBaseBehaviour arcameraBehaviour, TargetAbstractBehaviour targetBehaviour, Target target)
{
Debug.Log("<Global Handler> Lost: " + target.Id);
}
void OnTargetLoad(ImageTrackerBaseBehaviour trackerBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target, bool status)
{
Debug.Log("<Global Handler> Load target (" + status + "): " + target.Id + " (" + target.Name + ") " + " -> " + trackerBehaviour);
}
void OnTargetUnload(ImageTrackerBaseBehaviour trackerBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target, bool status)
{
Debug.Log("<Global Handler> Unload target (" + status + "): " + target.Id + " (" + target.Name + ") " + " -> " + trackerBehaviour);
}
}
Target Show/Hide¶
From EasyAR SDK 2.0, the target show/hide behaviour is default turn on. You can turn it off by Uncheck GameObject Active Control on Target Inspector. You can find this option on all target types. You can also turn it off in the script by setting TargetAbstractBehaviour.GameObjectActiveControl to false.
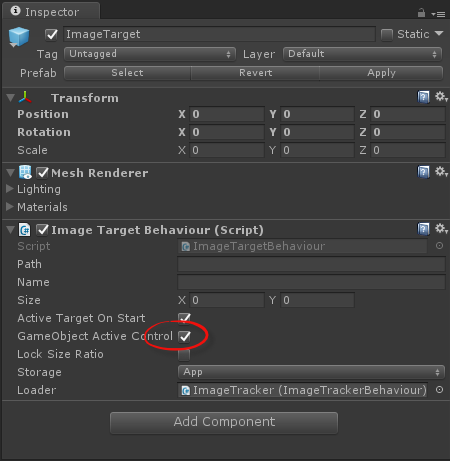
The default behaviour of GameObjectActiveControl is,
protected virtual void Start()
{
if (gameObjectActiveControl)
gameObject.SetActive(false);
}
internal void OnTargetFound()
{
if (gameObjectActiveControl)
gameObject.SetActive(true);
}
internal void OnTargetLost()
{
if (gameObjectActiveControl)
gameObject.SetActive(false);
}
This is a piece of internal code, it just shows what EasyAR SDK is doing when GameObjectActiveControl is on, and it will not work in your code even if you paste it in your projects. If you need to handle more complicated case, follow instructions bellow.
So if you turn GameObjectActiveControl off, you can make things change as you like and gain more freedom of target show/hide control, by overwrite Start and implement OnTargetFound/OnTargetLost event handling. More specifically, you can achieve the internal behaviour when GameObjectActiveControl is off as below, which is quite similar of doing so in EasyAR SDK 1.x,
public class EasyImageTargetBehaviour : ImageTargetBehaviour
{
protected override void Awake()
{
base.Awake();
GameObjectActiveControl = false;
TargetFound += OnTargetFound;
TargetLost += OnTargetLost;
}
protected override void Start()
{
base.Start();
gameObject.SetActive(false);
}
void OnTargetFound(TargetAbstractBehaviour behaviour)
{
gameObject.SetActive(true);
}
void OnTargetLost(TargetAbstractBehaviour behaviour)
{
gameObject.SetActive(false);
}
}
Bundle ID (Android/iOS)¶
You need to set bundle ID when generating Android/iOS apps. The bundle ID should match the ID where the key is generated in the EasyAR website. Otherwise the initialize will fail. One exception is for Mac or Windows; such ID matches are not required on these two platforms.
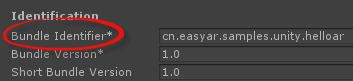
Graphics API (Android/iOS)¶
Set graphics API to OpenGL ES 2.0 if you are building Android or iOS apps. This setting is different across Unity version.
Unity 4.x settings are like this
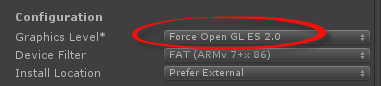

Unity 5.x settings are
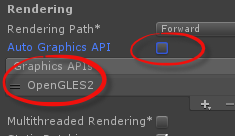
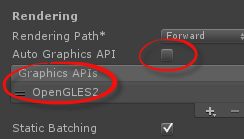
XCode configure (iOS)¶
If you are using latest Unity, this step is automatically done by Unity.
When you are generating iOS apps, after the automatic build step which put everything from Unity to XCode, you need one more step to make all things work.
XCode 6.x: add libc++.dylib into linker libraries.
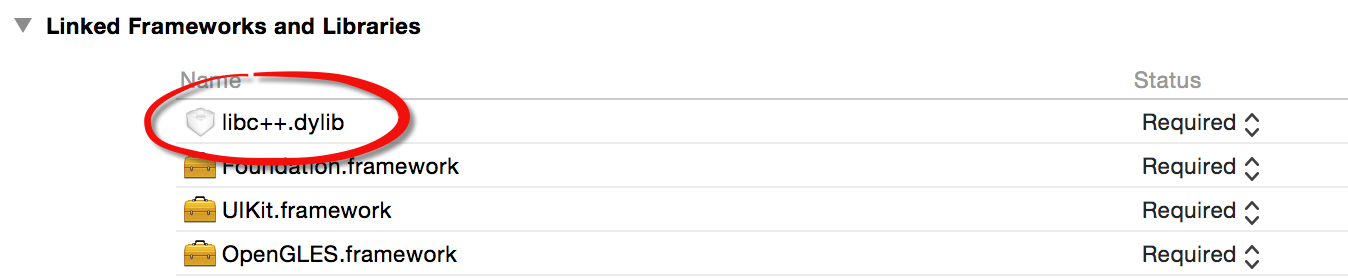
XCode 7.x: add libc++.tbd into linker libraries. And Set Enable Bitcode to NO .

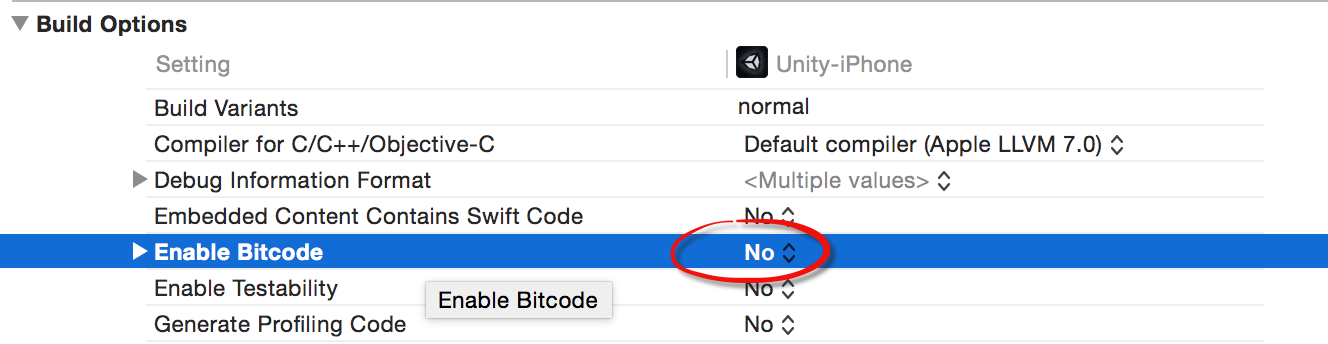
XCode configure for EasyAR SDK Pro¶
Add Accelerate.framework if you are using EasyAR SDK Pro samples.

iOS Privacy Settings¶
Some privacy settings are required on iOS.
To use camera, add Privacy - Camera Usage Description,

To use screen recording feature, add Privacy - Microphone Usage Description,

iOS arm64 Support¶
Turn on IL2CPP in Player Settings.
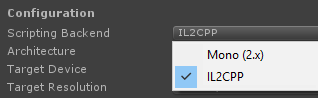
And change the architecture to Universal or arm64,
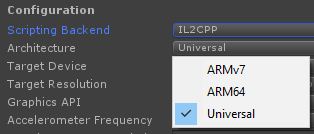
If you are using some older version of Unity, you may meet this compile error in XCode,

It is a Unity bug fixed in newer versions of Unity. It is suggested to upgrade your Unity, or just remove the word NORETURN
like this.

Android IL2CPP¶
IL2CPP support for Android is not included in the official EasyAR Unity Plugin, we will add support in later versions. But if you are writing your own EasyAR Plugin for Unity, you can always enable IL2CPP support.