MotionTracking¶
Demonstrate how to use motion tracking.
Demonstrate how to fallback to ARKit/ARCore/AREngine when EasyAR motion tracker not available
Reference: Motion Tracking and EasyAR Features.
How to Use¶
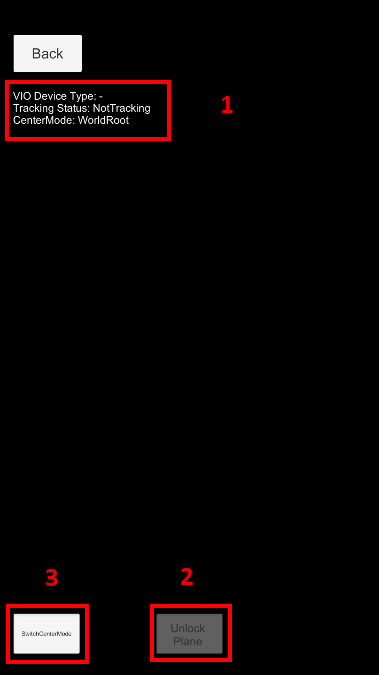
If EasyAR motion tracker is selected in runtime, the sample will detect horizontal plane at beginning. Plane detection will be stopped when then cube is placed on a plane. You can click on the Unlock Plane button to continue detect a new plane.
The cube in the scene can be moved on the plane with one finger. Two finger pinch will scale the cube and two finger horizontal move will rotate the cube.
If other frame source is selected in runtime, the sample will not detect horizontal plane.
How It Works¶
Strategy of frame source selection¶
The first available frame source in Transform order will be selected. You can adjust the order of frame source to change the selection order.
In this sample, EasyAR motion tracker will be used if it is available on device. Otherwise the first available one will be choosed in the order of AREngin, ARCore, ARKit.
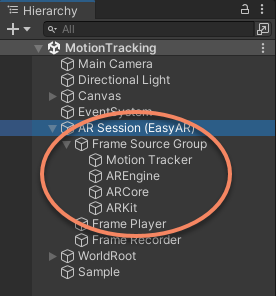
Error message will popup if all frame sources listed are not available on device,
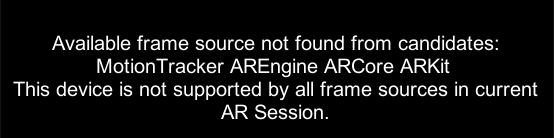
This error message does not always mean the device is not supported by EasyAR. Strictly, it means there is no frame source available from all frame sources for chosen under ARSession for the device. There are usually two possibilities,
The device is not supported by EasyAR, for example, when motion tracking is desired on Windows using external usb camera.
The available frame source is not listed under and chosable by ARSession. For example, when you run on iPhone which is ARKit ready but ARKit under ARSession is deleted.
Objects under world root¶
WorldRoot is designed to do these things,
Control show/hide of objects when tracking status change.
Move together against camera according to ARSession.CenterMode.
You can ignore WorldRoot If you can make sure all above is handled by yourself.
In this sample, WorldRootController.ActiveControl is set to ActiveControlStrategy.HideWhenNotTracking, so the cube will hide when tracking fails.
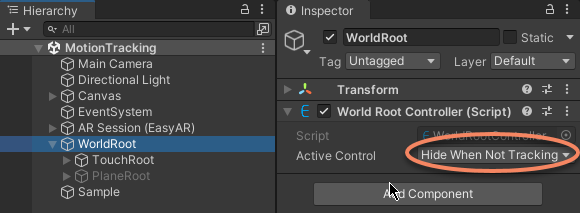
Plane detection¶
This part is only available when EasyAR motion tracking is running.
MotionTrackerFrameFilter.HitTestAgainstHorizontalPlane is used to get a plane location in the world. Place a plane at that location.
var viewPoint = new Vector2(0.5f, 0.333f);
var points = motionTracker.HitTestAgainstHorizontalPlane(viewPoint);
if (points.Count > 0)
{
Plane.transform.position = points[0];
...
}
Keep the plane when a plane is detected until it is about to go beyond sight.
var viewportPoint = Session.Assembly.Camera.WorldToViewportPoint(Plane.transform.position);
if (!Plane.activeSelf || viewportPoint.x < 0 || viewportPoint.x > 1 || viewportPoint.y < 0 || viewportPoint.y > 1 || Mathf.Abs(Plane.transform.position.y - points[0].y) > 0.15)
{
...
}
Move object on the plane¶
This part is only available when EasyAR motion tracking is running.
Raycast against the plane object and move the cube on it.
Ray ray = Session.Assembly.Camera.ScreenPointToRay(touch.position);
RaycastHit hitInfo;
if (Physics.Raycast(ray, out hitInfo))
{
TouchControl.transform.position = hitInfo.point;
...
}
Center mode¶
In ARSession.ARCenterMode.SessionOrigin the camera will move automatically when the device moves, and the WorldRoot stay. In ARSession.ARCenterMode.Camera, the camera do not automatically move when the device moves. For other center modes, please reference Center mode.