ObjectTracking¶
Demonstrate how to track 3D objects.
How to Use¶
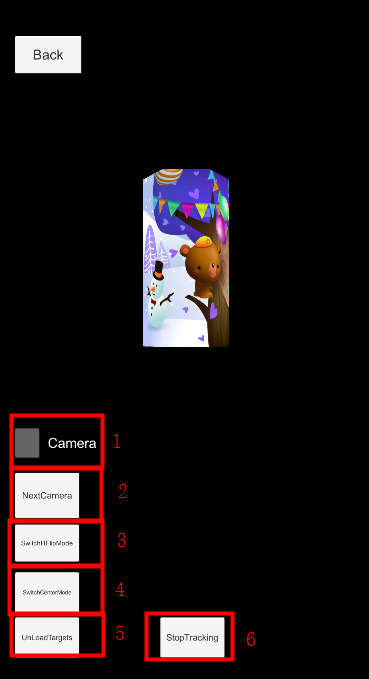
The object could be tracked is the same target in the scene. Find model texture image in the project, print out and make a hexagon from it.
How It Works¶
Targets in the scene¶
If you want to replace the model in the sample to your own, make sure to choose a .obj file with rich texture, and fill in Extra File Paths with all .mtl and .jpg/.png paths referenced in .obj and .mtl files. It is also suggested to read this guide to help choosing a trackable object.
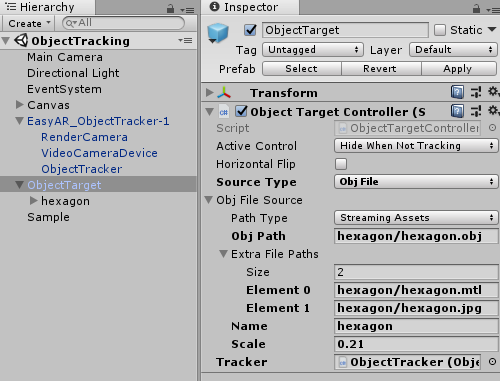
Target can be setup directly from the inspector of Unity editor.
Active Control: Targets and their children will hide when the target is not being tracked. If you need to keep it display when lost, change this value and write your own strategy.
Source Type: Targets are created from Obj File in this sample.
Path Type: StreamingAssets here, so that all the Path will use a path relative to StreamingAssets.
Obj Path: .obj file path relative to StreamingAssets in this sample.
Extra File Paths: .mtl and .jpg file path relative to StreamingAssets in this sample.
Name: Name of the target, choose a world easy to remember.
Scale: It is set according to physical size of the hexagon foldered using A4 size paper in real world.
Tracker: The Tracker to load ObjectTarget.
Gizmo is displayed after load if not disabled in the global settings when the target setting is valid. This will not show in the game view.
The transform of the hexagon has been adjusted so that it will be aligned with the target. When you run this scene and track the target, the hexagon will show and cover the target.
Target events¶
Target events are used for custom operations, the sample use the events to output some logs. You can delete the logs if they are not used, and you can use the events to handle your game logic.
controller.TargetFound += () =>
{
Debug.LogFormat("Found target {{id = {0}, name = {1}}}", controller.Target.runtimeID(), controller.Target.name());
};
controller.TargetLost += () =>
{
Debug.LogFormat("Lost target {{id = {0}, name = {1}}}", controller.Target.runtimeID(), controller.Target.name());
};
controller.TargetLoad += (Target target, bool status) =>
{
Debug.LogFormat("Load target {{id = {0}, name = {1}}} into {2} => {3}", target.runtimeID(), target.name(), controller.Tracker.name, status);
};
controller.TargetUnload += (Target target, bool status) =>
{
Debug.LogFormat("Unload target {{id = {0}, name = {1}}} => {2}", target.runtimeID(), target.name(), status);
};
Target load and unload¶
Target load and unload is very easy. Set ObjectTargetController.Tracker to null, the target will be unloaded, and set it to some tracker will make the target loaded into the tracker immediately.
public void UnloadTargets()
{
objectTarget.Tracker = null;
}
public void LoadTargets()
{
objectTarget.Tracker = objectTracker;
}
Tracking on/off¶
Object tracking can be turned on or off using ObjectTrackerFrameFilter.enabled. You can turn the tracking off when it is not used to save performance, it will not turn off the camera or any other tracking features.
public void Tracking(bool on)
{
objectTracker.enabled = on;
}
Camera on/off¶
Camera device can be turned on or off using VideoCameraDevice.enabled. If a camera has turned off, the tracker will not receive any frames and the whole AR chain will stop.
public void EnableCamera(bool enable)
{
cameraDevice.enabled = enable;
}
Center mode¶
Three mode of ARSession.CenterMode are valid in object sensing. See detailed description in Center mode.
Flip camera image horizontally¶
ARSession.HorizontalFlipNormal and ARSession.HorizontalFlipFront controls how the camera is mirrored. When the camera image is mirrored, the camera projection or the target scale will change so that a tracking behavior continues. See detailed description in Flip camera image horizontally.