ImageTracking_TargetOnTheFly¶
Demonstrate how to create image target directly from real-time camera image and load it into tracker as a target.
How to Use¶
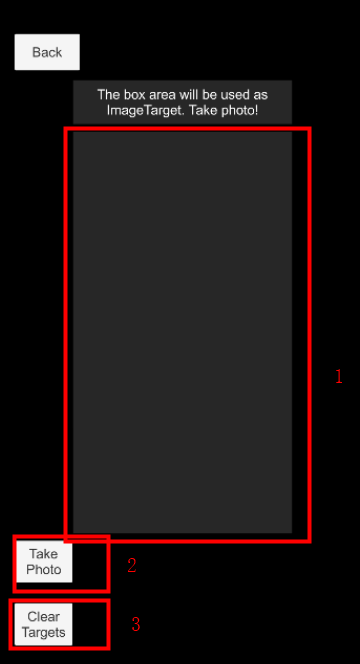
Mark 1: Region for image capture.
Mark 2: Capture image in mark 1 to create image target.
Mark 3: Unload and delete all image targets and caches.
How It Works¶
Use part of camera image as target¶
This sample use part of the camera image as target. First get that data and encode to image.
private IEnumerator TakePhotoCreateTarget()
{
...
yield return new WaitForEndOfFrame();
...
Texture2D photo = new Texture2D(Screen.width / 2, Screen.height / 2, TextureFormat.RGB24, false);
photo.ReadPixels(new Rect(Screen.width / 5, Screen.height / 5, Screen.width * 3 / 5, Screen.height * 3 / 6), 0, 0, false);
photo.Apply();
byte[] data = photo.EncodeToJPG(80);
Destroy(photo);
string photoName = "photo" + DateTime.Now.Ticks + ".jpg";
string photoPath = Path.Combine(directory, photoName);
File.WriteAllBytes(photoPath, data);
CreateImageTarget(photoName, photoPath);
...
}
You can then create targets from images. Create an empty GameObject and add ImageTargetController and setup the ImageTargetController with image file.
private void CreateImageTarget(string targetName, string targetPath)
{
GameObject imageTarget = new GameObject(targetName);
var controller = imageTarget.AddComponent<ImageTargetController>();
controller.SourceType = ImageTargetController.DataSource.ImageFile;
controller.ImageFileSource.PathType = PathType.Absolute;
controller.ImageFileSource.Path = targetPath;
controller.ImageFileSource.Name = targetName;
controller.Tracker = Filter;
...
}
This sample use a direct way to handle target creation, but it is not in the best performance. You can also create an Image with image data byte array, and create target directly using ImageTarget.createFromParameters to achieve better performance.