Setting up EasyAR Unity SDK¶
This article shows how to setup EasyAR Unity projects using EasyAR.unitypackage.
If you want to run EasyAR unity sample, please read this instead.
Pre-Requirements¶
Unity 4.6 or later
(If target for Android) Android SDK with Build Tools at least version 20.0.0
(If target for iOS) IPhone or IPad device, or other real Apple devices (EasyAR do not support running on the simulator)
Import Package¶
First, you have to download EasyAR package, find EasyAR.unitypackage and open to import it into Unity.
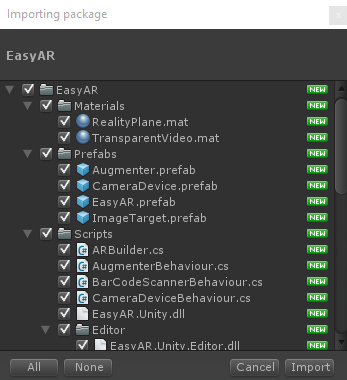
Initialize EasyAR¶
To make EasyAR work, you have to have EasyAR prefab or other prefabs in the scene. Drag EasyAR Prefab into the scene.
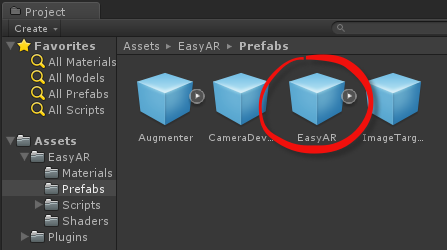
You can create a key after login EasyAR website. You can find how to create the key from Getting Started with EasyAR SDK.
Next you need to add two lines into your initialize code to initialize EasyAR with your key. (until 1.2.1)
ARBuilder.Instance.InitializeEasyAR(key);
ARBuilder.Instance.EasyBuild();
If you use the default configure (CameraDeviceBaseBehaviour.CaptureWhenStart is enabled), EasyAR will begine to run at MonoBehaviour.Start. So it is better to put above code into Awake.
If you want to see the same input text area like the samples, you can create a script and write the following code. Then drag the script onto the EasyAR prefab. (until 1.2.1)
using UnityEngine;
namespace EasyAR
{
public class ARIsEasyBehaviour : MonoBehaviour
{
[TextArea(1, 10)]
public string Key;
private void Awake()
{
ARBuilder.Instance.InitializeEasyAR(Key);
ARBuilder.Instance.EasyBuild();
}
}
}
In the EasyAR 1.3 or later, you just need to past the key into the EasyAR_xxx object. There is a default script attached to the object already. (since 1.3.0)
Add ImageTarget¶
There are many ways to use the ImageTarget. You can reference the HelloARTarget sample.
If you want to setup ImageTarget statically in the scene, you have to drag an ImageTarget Prefab into the scene. Read ImageTarget Prefab and ImageTargetBaseBehaviour for details about configurations.
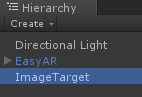
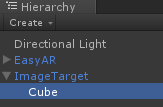
Target Events (until 1.2.1)¶
You can handle target event either in ImageTargetBehaviour
public class EasyImageTargetBehaviour : ImageTargetBehaviour, ITargetEventHandler
{
void ITargetEventHandler.OnTargetFound(Target target)
{
Debug.Log("Found: " + target.Id);
}
void ITargetEventHandler.OnTargetLost(Target target)
{
Debug.Log("Lost: " + target.Id);
}
void ITargetEventHandler.OnTargetLoad(Target target, bool status)
{
Debug.Log("Load target (" + status + "): " + target.Id + " -> " + target.Name);
}
void ITargetEventHandler.OnTargetUnload(Target target, bool status)
{
Debug.Log("Unload target (" + status + "): " + target.Id + " -> " + target.Name);
}
}
or in a global target manager that implements ITargetEventHandler
public class EasyARTargetMananger : MonoBehaviour, ITargetEventHandler
{
void ITargetEventHandler.OnTargetFound(Target target)
{
Debug.Log("Found: " + target.Id);
}
void ITargetEventHandler.OnTargetLost(Target target)
{
Debug.Log("Lost: " + target.Id);
}
void ITargetEventHandler.OnTargetLoad(Target target, bool status)
{
Debug.Log("Load target (" + status + "): " + target.Id + " -> " + target.Name);
}
void ITargetEventHandler.OnTargetUnload(Target target, bool status)
{
Debug.Log("Unload target (" + status + "): " + target.Id + " -> " + target.Name);
}
}
You can show/hide objects under ImageTarget in the target events.
Target Events (since 1.3.0)¶
You can handle target event either in ImageTargetBehaviour
public class EasyImageTargetBehaviour : ImageTargetBehaviour
{
protected override void Awake()
{
base.Awake();
TargetFound += OnTargetFound;
TargetLost += OnTargetLost;
TargetLoad += OnTargetLoad;
TargetUnload += OnTargetUnload;
}
void OnTargetFound(ImageTargetBaseBehaviour behaviour)
{
Debug.Log("Found: " + Target.Id);
}
void OnTargetLost(ImageTargetBaseBehaviour behaviour)
{
Debug.Log("Lost: " + Target.Id);
}
void OnTargetLoad(ImageTargetBaseBehaviour behaviour, ImageTrackerBaseBehaviour tracker, bool status)
{
Debug.Log("Load target (" + status + "): " + Target.Id + " (" + Target.Name + ") " + " -> " + tracker);
}
void OnTargetUnload(ImageTargetBaseBehaviour behaviour, ImageTrackerBaseBehaviour tracker, bool status)
{
Debug.Log("Unload target (" + status + "): " + Target.Id + " (" + Target.Name + ") " + " -> " + tracker);
}
}
or in a global target manager
public class EasyARTargetMananger : MonoBehaviour
{
private void Awake()
{
FindObjectOfType().Initialize();
foreach (var behaviour in ARBuilder.Instance.AugmenterBehaviours)
{
behaviour.TargetFound += OnTargetFound;
behaviour.TargetLost += OnTargetLost;
behaviour.TextMessage += OnTextMessage;
}
foreach (var behaviour in ARBuilder.Instance.TrackerBehaviours)
{
behaviour.TargetLoad += OnTargetLoad;
behaviour.TargetUnload += OnTargetUnload;
}
}
void OnTargetFound(AugmenterBaseBehaviour augmenterBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target)
{
Debug.Log("Found: " + target.Id);
}
void OnTargetLost(AugmenterBaseBehaviour augmenterBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target)
{
Debug.Log("Lost: " + target.Id);
}
void OnTargetLoad(ImageTrackerBaseBehaviour trackerBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target, bool status)
{
Debug.Log("Load target (" + status + "): " + target.Id + " (" + target.Name + ") " + " -> " + trackerBehaviour);
}
void OnTargetUnload(ImageTrackerBaseBehaviour trackerBehaviour, ImageTargetBaseBehaviour targetBehaviour, Target target, bool status)
{
Debug.Log("Unload target (" + status + "): " + target.Id + " (" + target.Name + ") " + " -> " + trackerBehaviour);
}
private void OnTextMessage(AugmenterBaseBehaviour augmenterBehaviour, string text)
{
Debug.Log("got text: " + text);
}
}
You can show/hide objects under ImageTarget in the target events.
Bundle ID (Android/iOS)¶
You need to set bundle ID when generating Android/iOS apps. The bundle ID should match the ID where the key is generated in the EasyAR website. Otherwise the initialize will fail. One exception is for Mac or Windows; such ID matches are not required on these two platforms.
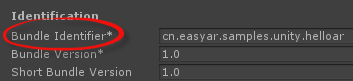
Graphics API (Android/iOS)¶
Set graphics API to OpenGL ES 2.0 if you are building Android or iOS apps. This setting is different across Unity version.
Unity 4.x settings are like this
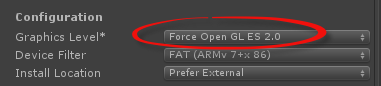

Unity 5.x settings are
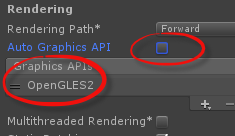
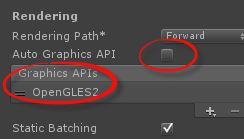
XCode configure (iOS)¶
If you are using latest Unity, this step is automatically done by Unity.
When you are generating iOS apps, after the automatic build step which put everything from Unity to XCode, you need one more step to make all things work.
XCode 6.x: add libc++.dylib into linker libraries.
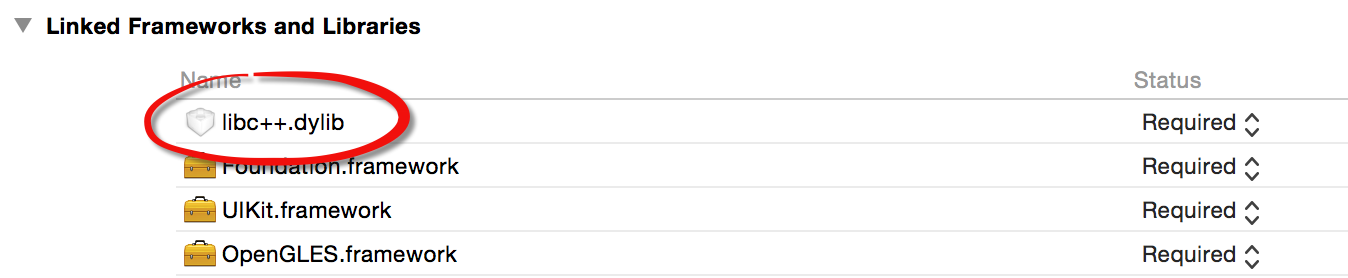
XCode 7.x: add libc++.tbd into linker libraries. And Set Enable Bitcode to NO .

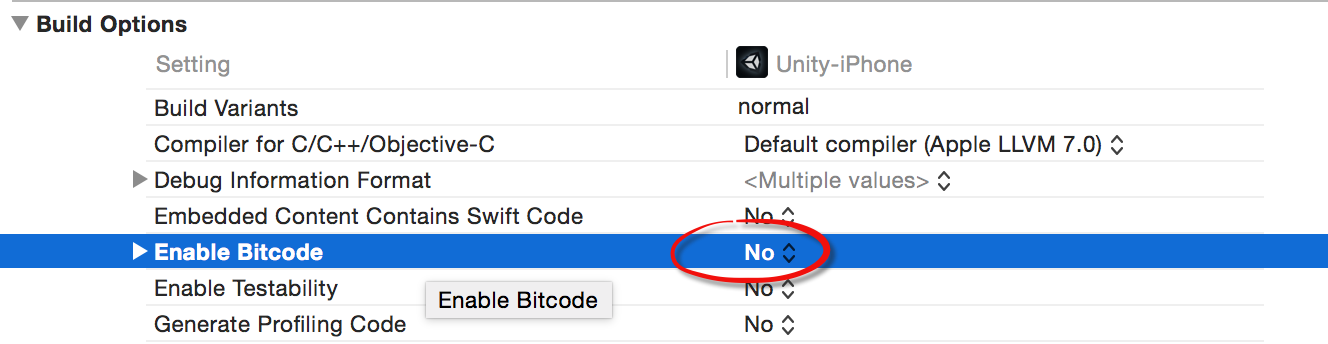