VideoRecording¶
Demonstrate how to do recording.
How to Use¶
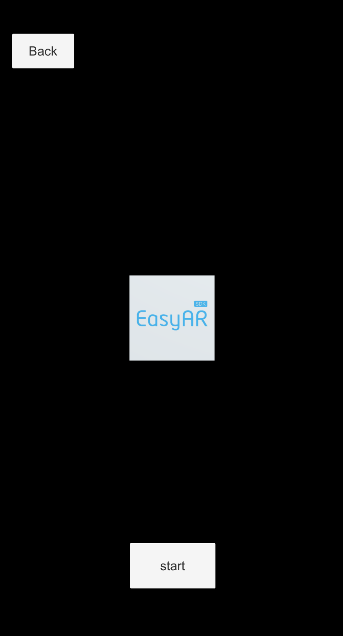
Start/Stop: Start/Stop recording.
How It Works¶
Recorder in the scene¶
You need to add a VideoRecorder in the scene to record video. You can reference API documents in the scripts for detailed settings.
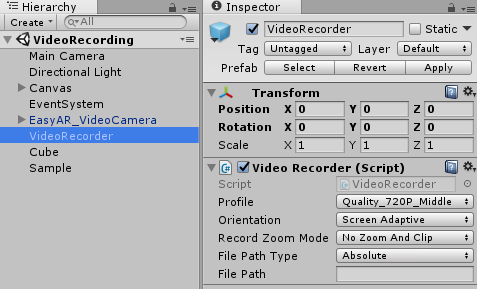
Record camera output¶
To record the camera output, just add a Component to the camera.
cameraRecorder = Camera.main.gameObject.AddComponent<CameraRecorder>();
And call VideoRecorder.RecordFrame in OnRenderImage.
public void OnRenderImage(RenderTexture source, RenderTexture destination)
{
Graphics.Blit(source, destination);
...
videoRecorder.RecordFrame(source);
}
Record camera output with overlay¶
If you want to add some overlay to the video or make other effects, a Graphics.Blit or other methods before RecordFrame would do it.
public void OnRenderImage(RenderTexture source, RenderTexture destination)
{
Graphics.Blit(source, destination);
...
Graphics.Blit(source, rt, externalMaterial);
videoRecorder.RecordFrame(rt);
}
Recorder events¶
VideoRecorder.StatusUpdate can be used to get recorder status.
videoRecorder.StatusUpdate += (status, msg) =>
{
if (status == RecordStatus.OnStarted)
{
GUIPopup.EnqueueMessage("Recording start", 5);
}
if (status == RecordStatus.FailedToStart || status == RecordStatus.FileFailed || status == RecordStatus.LogError)
{
GUIPopup.EnqueueMessage("Recording Error: " + status + ", details: " + msg, 5);
}
Debug.Log("RecordStatus: " + status + ", details: " + msg);
};
What can be recorded¶
This sample record the camera output. But that is not all the VideoRecorder can do. VideoRecorder.RecordFrame accept any RenderTexture feed into it. The recorded texture is passed by the user code, so you have full control of what is recorded.